Developing Secure Software for Autonomous Vehicles
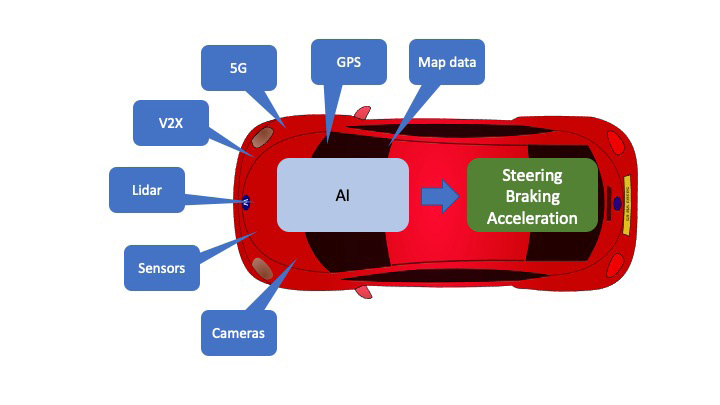
Autonomous vehicles and the need for cybersecurity
The automotive industry is going through a transformation and moving toward software-defined vehicles where a majority of functionality is provided and controlled by software. This allows for updatability, scalability and flexibility, which leads to improved user experience. One such trending functionality is autonomous driving functionality. The automotive industry is working on several standardization activities to guide automotive organizations develop safe and secure autonomous vehicles (AVs). For example, a set of updated provisional national standards to guide the deployment of safe and secure AVs in Singapore was released in August this year. Known as TR68 [1], this technical reference comprises four parts and is a comprehensive guide for both AV developers and AV operators. The first part covers basic behavior of AVs, including topics on Dynamic Driving Task (DDT) and Automated Driving System (ADS). The second part is on safety guidance for AVs including design, production, and safe operation. The third part covers cybersecurity, focusing on high-level security principles and a cybersecurity assessment framework. The fourth part is on vehicular data types and formats related to data recorded for automated driving, HD mapping, and V2X information exchange.
It is important to recognize that while AVs provide many benefits, there are serious cybersecurity risks that need to be considered. For instance, a semi-autonomous vehicle can contain more than 300 million lines of code, and it is estimated that a fully autonomous car will contain more than 1 billion lines of code. The development of new, complex and large software solutions combined with the use of novel and advanced technologies and interfaces such as Artificial Intelligence (AI), Lidar, sensors, cameras, V2X, and 5G increase the risk for introduction of vulnerabilities. As a simplified example, AVs use sensors and cameras to gather input about their surroundings together with GPS and map data. This input is then processed by AI to make decisions that control steering, braking and acceleration of the vehicle, as shown in Figure 1. Cyberattackers can deliberately target these different attack surfaces to cause the AV to misbehave, which could have fatal consequences.
Automotive organizations becoming software organizations
With the shift toward software-defined vehicles, autonomous driving functionality could be considered as one application providing a set of functionality running on top of a software platform or operating system (OS) in the vehicle. The development of these types of applications and software platforms is causing a disruption in the automotive industry leading to a supply chain transformation. That is, more automotive software is developed to be run on platforms, different software variants are being consolidated, and software is becoming independent from hardware, which allows reuse of software components. Automotive organizations are also acquiring or partnering with tech companies, including cloud providers, software development service providers and cybersecurity companies. Figure 2 provides an overview of an HPC (high-performance computer) architecture, where different OS are running on top of a hypervisor. For example, the autonomous driving functionality could be provided by an application running on the AUTOSAR Adaptive Platform. In addition, a Trusted Execution Environment, with a root of trust in hardware [3] can provide a number of security features including secure boot, secure execution and secure storage.
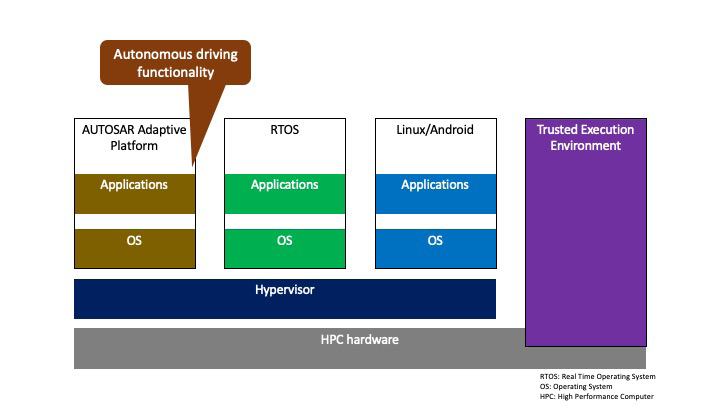
For automotive organizations to be able to more efficiently develop these types of software, there is a need to shift the development methodology from the traditional V-model often used for ECU (electronic control unit) software development to a more agile DevSecOps development methodology. Adopting a DevSecOps methodology allows the organization to improve development speed, reduce costs, perform automated testing through the use of appropriate tools, and improve quality and security through continuous feedback. Depending on the type of software, it is possible to perform various activities for continuous integration, continuous testing and continuous delivery as shown in Figure 3.
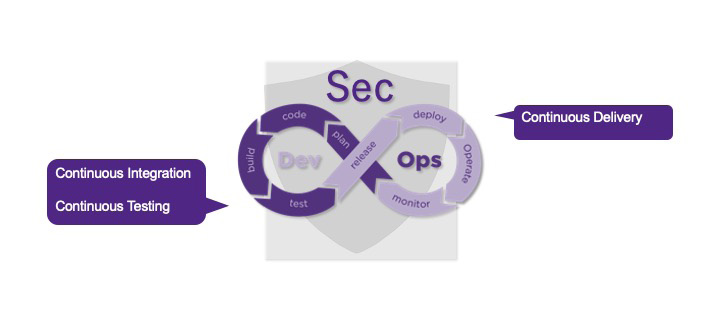
To change the software development methodology, the automotive industry can learn from other industries that have already started this journey. For example, there was a study conducted of 440 large software development organizations, across 12 industries and nine countries, that measured 46 activities related to software development with the goal to identify the key success factors [4]. The top most important items of the measured activities were grouped into four groups, i.e., the four key success factors for software development: development tools, talent management, culture and product management. This article focuses on solutions for development tools.
Application security testing approaches
As mentioned, deploying the right development tools is one of the top items identified as a success factor for software development. To get a better perspective of DevSecOps, a study of 350 enterprise IT organizations was conducted with the goal to identify the most critical application security testing to add to their DevSecOps program [5]. An overview of the results from the study is presented in Figure 4.
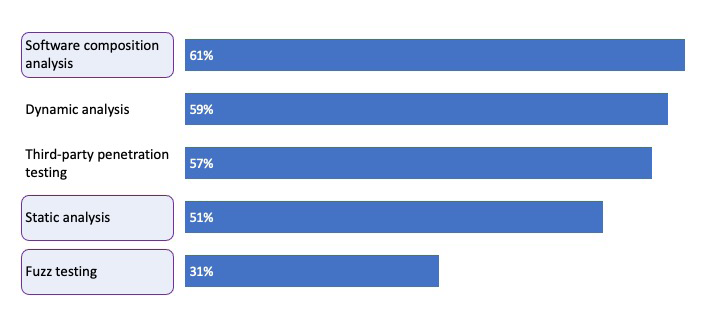
In the following, we discuss software composition analysis, static analysis and fuzz testing related to AVs in more detail, since there exist appropriate automated tools that can be deployed. While dynamic analysis and third-party penetration testing are also included in the top results from the study, the main goal with DevSecOps is to use automated tools, and there are currently not many automated tools for these test approaches applicable to AVs.
Regarding software composition analysis, the purpose is to identify included open-source software components in the codebase for the automotive system, as well as further identify any associated known vulnerabilities in the included open-source software components. First, it is important to recognize that there may be open-source software components included in the autonomous driving system. For example, the Autoware Foundation supports open-source projects for self-driving mobility, including functions for autonomous valet parking [6]. There may also be various open-source communication stacks or libraries used in the autonomous driving system providing commonly used functionality. Using a software composition analysis tool integrated into the DevSecOps process allows for automated detection of included open-source software components, associated known vulnerabilities, and continuous vulnerability monitoring even after software has been released [7].
Regarding static analysis, there are automated tools that can scan source code to detect various defects such as buffer overflows, resource leaks, memory corruption, hard-coded credentials, null pointer deference etc. [8]. Equally important for safety-critical systems, is the need to check for compliance to certain coding guidelines such as MISRA C/C++ and AUTOSAR C++, which can be achieved using static analysis tools. More specifically, for autonomous driving systems, CUDA is often used, and as such, it is imperative that the static analysis tool supports checkers for CUDA. CUDA stands for Compute Unified Device Architecture and is a general-purpose parallel computing platform and programming model for GPUs developed by NVIDIA. There is a dedicated C/C++ compiler (nvcc) and libraries (API) available. CUDA’s main purpose is to accelerate compute-intensive applications by leveraging GPUs for parallel processing. There are already more than 25 companies using NVIDIA CUDA GPU to develop fully autonomous robot taxis (SAE Level 5).
A simplified example of CUDA processing flow is illustrated in Figure 5 [9]. In the center of the figure is the application code. Part of the application code contains compute-intensive functions, highlighted by the gray box, that will be executed on the Device (GPU). The rest of the code will be executed on the Host (CPU). There are four steps in the CUDA processing flow. In the first step, the host will copy the data that will be used for the compute-intensive function from the Host Memory to the Device Memory. At the second step, the Host instructs the Device to execute the compute-intensive function. In the third step, the Device will process the compute-intensive function in parallel on multiple cores, reading the stored data from Device Memory from step 1 and storing the resulting output back into Device Memory. Finally, as the fourth step, the output stored in Device Memory is copied back to the Host Memory and can then be used by the rest of the application code on the Host. This type of processing flow is useful for autonomous driving applications using compute-intensive functions such as image processing that can be offloaded to the Device and processed in parallel on multiple cores on the GPU.
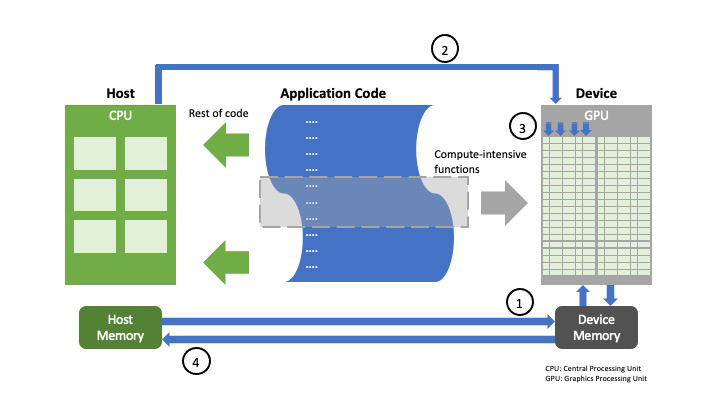
The following code example provides some more specifics on CUDA extensions used in C programming language [9, 10]. To better understand the example, some CUDA relevant terminology is described first.
kernel : code that will execute on the Device
__global__ : declares a function called from Host (CPU) and executed on Device (GPU)
<<< >>>() : function call from Host to a kernel on the Device
To support memory management of the Device Memory, the following functions are available:
cudaMalloc()
cudaMemcpy()
cudaFree()
The code example in Figure 6 is described as follows, highlighting the CUDA relevant parts. First, ___global___ is used to declare that the add() function will be called from the Host and executed on the Device. Then, cudaMalloc() is used to allocate space on the Device Memory to be able to store the data used as input. Next, cudaMemcpy() is called to copy the data used as input from Host Memory to Device Memory. Using the copied data as input, the add() kernel is executed on the Device by calling it using <<< >>>(). Once the Device has processed the input and stored the result on Device Memory, the result is copied to the Host Memory using cudaMemcpy(). Finally, the Device Memory is cleaned up using cudaFree(). This simple example illustrates code executed on both Host and Device and the need for memory management. To help developers avoid programming mistakes, static analysis can be integrated into the DevSecOps process to run CUDA specific checkers that can detect issues with, e.g., kernel launch, fork, device threads, synchronization and memory management [8, 11].
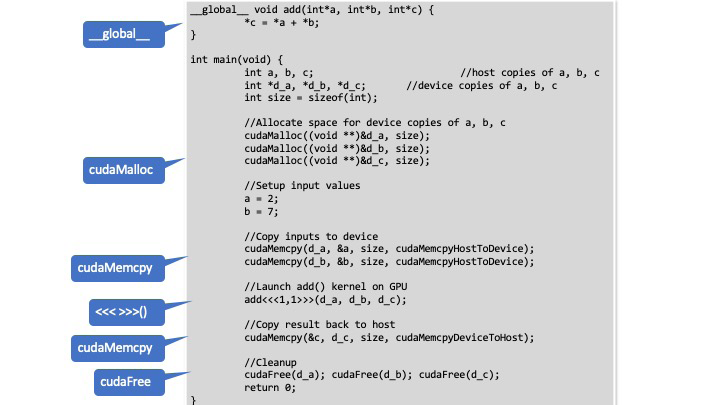
Regarding fuzz testing, there are automated tools that can generate invalid input that is then provided to a target system to detect unknown vulnerabilities. For example, fuzz testing tools can be used to test protocol implementations of among other CAN, Ethernet, Wi-Fi, and Bluetooth [12]. Fuzz testing can also be integrated into the DevSecOps process to automate testing [13, 14]. More specifically for AVs, C-V2X communication based on 5G is becoming more relevant. 5G Automotive Association (5GAA) is a global, cross-industry organization of companies that was created in 2016 to connect the telecom industry and vehicle manufacturers to develop end-to-end solutions for future mobility and transportation services. 5GAA describes a number of C-V2X use cases including cross-traffic left-turn assist, intersection movement assist, emergency brake warning, traffic jam warning, speed harmonization, and lane change warning [15]. While it is important to test the external interfaces on AVs, it is equally important to test the security and robustness of the 5G network supporting AVs through these types of use cases. The 5G network is a complex interconnected system comprising a set of different components and protocols as illustrated in Figure 7.
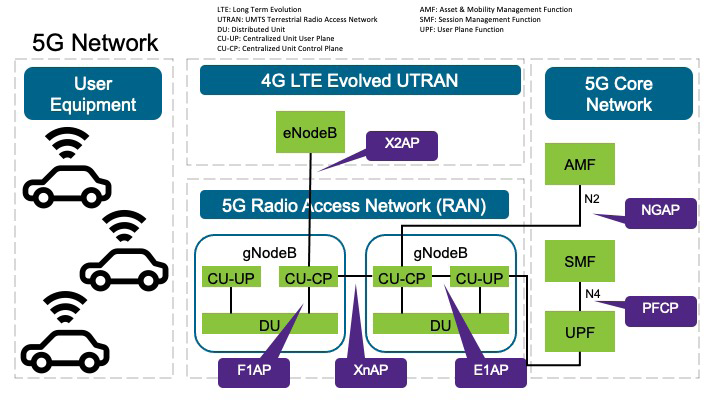
For example, AVs are considered user equipment in this network. Moreover, there is communication between 4G eNodeB base stations and 5G gNodeB base stations using X2AP protocol, communication between 5G gNodeB base stations using XnAP protocol, communication within 5G gNodeB base stations using F1AP and E1AP protocols, communication between 5G gNodeB base stations and 5G core network using NGAP protocol, and communication within the 5G core network using PFCP protocol among others. Potential software vulnerabilities in any of these protocol implementations could potentially have impact on security, robustness and availability of features and functions required for successful execution of use cases to support AVs. Therefore, performing fuzz testing of such protocol implementations to detect unknown vulnerabilities play a major role in ensuring the robustness and security of the deployment of 5G networks.
Summary
With the development of more advanced autonomous driving functionality, including the usage of more external inputs and communications, larger software codebases and potential usage of open-source software, the need for cybersecurity becomes paramount. The automotive industry is seeing a shift toward DevSecOps development methodology to improve development speed, reduce costs, perform automated testing through the use of appropriate tools, and improve quality and security through continuous feedback. Specifically, this article highlights the usage of automated tools for software composition analysis, static analysis and fuzz testing to detect vulnerabilities earlier in the development lifecycle.
References
[1] Manufacturing Standards Committee, “TR 68”, 2021
[2] Dennis Kengo Oka, “Building Secure Cars: Assuring the Automotive Software Development Lifecycle”, Wiley, 2021
[3] Synopsys, “DesignWare tRoot Secure Hardware Root of Trust”, https://www.synopsys.com/dw/ipdir.php?ds=security-troot-hw-root-of-trust
[4] Georg Doll, “When things get complex”, Automotive Computing Conference 2020
[5] 451 Research Advisory, “DevSecOps Realities and Opportunities”, 2018
[6] The Autoware Foundation, https://www.autoware.org/
[7] Synopsys, “ Black Duck Software Composition Analysis”, https://www.synopsys.com/software-integrity/security-testing/software-composition-analysis.html
[8] Synopsys, “Coverity Static Application Security Testing”, https://www.synopsys.com/software-integrity/security-testing/static-analysis-sast.html
[9] NVIDIA, “CUDA C/C++ Basics”, https://www.nvidia.com/docs/IO/116711/sc11-cuda-c-basics.pdf
[10] NVIDIA, “CUDA Toolkit Documentation”, https://docs.nvidia.com/cuda/cuda-compiler-driver-nvcc/index.html
[11] NVIDIA, “CUDA C++ Programming Guide”, https://docs.nvidia.com/cuda/pdf/CUDA_C_Programming_Guide.pdf
[12] Synopsys, “ Defensics Fuzz Testing”, https://www.synopsys.com/software-integrity/security-testing/fuzz-testing.html
[13] Dennis Kengo Oka, and Tommi Makila, “A Practical Guide to Fuzz Testing Embedded Software in a CI Pipeline”, FISITA World Congress 2021
[14] Nico Vinzenz, and Dennis Kengo Oka, “Integrating Fuzz Testing into the Cybersecurity Validation Strategy,” SAE World Congress 2021, SAE Technical Paper 2021-01-0139, https://doi.org/10.4271/2021-01-0139
[15] 5GAA, “C-V2X Use Cases Methodology, Examples and Service Level Requirements”, White Paper
Author:
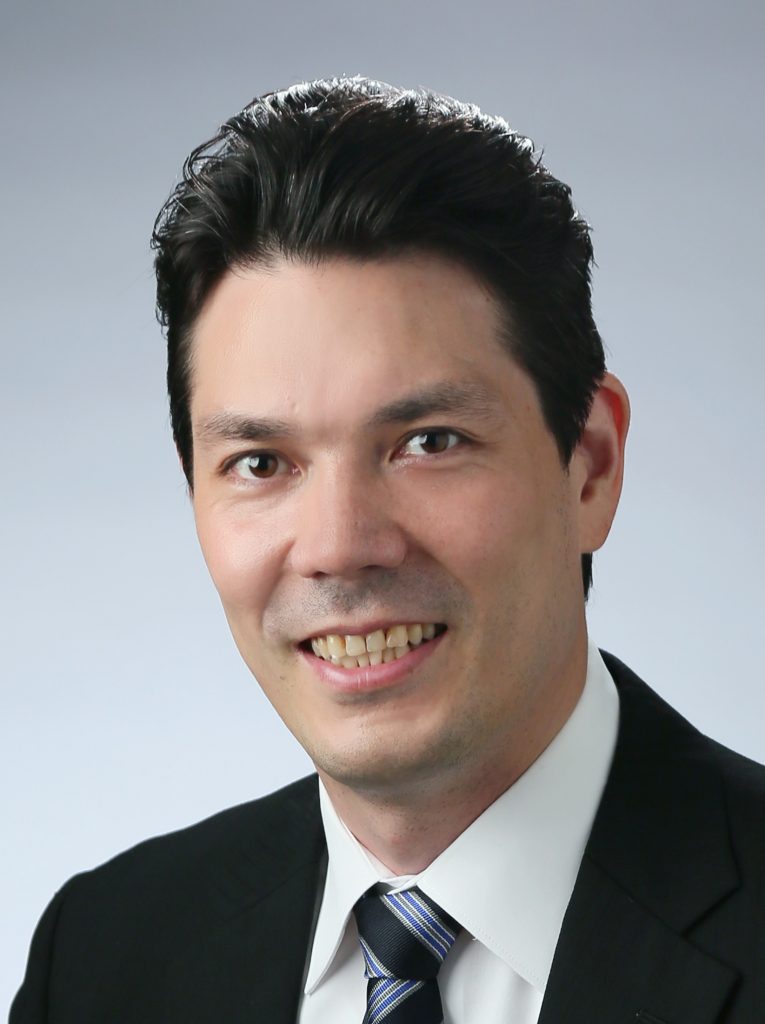
Dennis Kengo Oka
Principal Automotive Security Strategist
Synopsys
Dr. Dennis Kengo Oka is an automotive cybersecurity expert with more than 15 years of experience in the automotive industry. As a Principal Automotive Security Strategist at Synopsys, he focuses on security solutions for the automotive software development lifecycle and supply chain. Dennis has over 70 publications consisting of conference papers, articles and books, and is a frequent speaker at international automotive and cybersecurity conferences.
Published in Telematics Wire